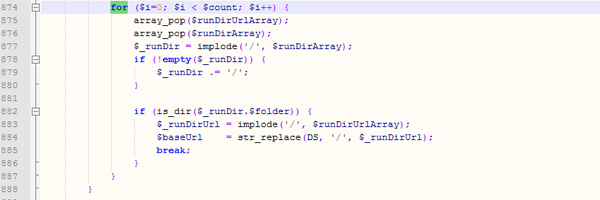
Programming is, and will probably always be an interest of mine. I know when I first started out, I had questions, many, many questions.
One question that used to boggle my mind is why have so many different control structures that do the same thing. In essences for, foreach and while can be used to do the same exact thing. They loop through things. Each control structures though, can perform faster depending on what logic you are trying to do.
FOR
Reference Material: http://php.net/manual/en/control-structures.for.php
It is best used for integer based loops. For example:
for ($i = 1; $i = 10; $i++) { echo $i; }
Output:
12345678910
What it is doing is supplying 3 expressions that you can set.
- Expression 1: This is executed at the beginning of the loop and is where you set variables. It is only executed once. In this example we set
$i
to equal1
- Expression 2: This is evaluated at the beginning of each loop(iteration) with the exception of the first. If it evaluates as
TRUE
, the loop continues and the nested statement(s) are executed. If it evaluates toFALSE
, the execution of the loop ends. If a nested statement executes abreak;
, the loop will end. In this example, we will loop while$i
is less than or equal to10
. Once$i
is greater than10
the loop will end. - Expression 3: This is executed at the end of each loop(iteration). In this example we executed this code
$i++
. We could have instead executedprint $i, $i++
and not have an echo statement as this will do the same thing.
Anytime I am working with a loop and integers, this is the loop I go with.
FOREACH
Reference Material: http://php.net/manual/en/control-structures.foreach.php
It is best used for objects or array based loops that are not from a database. For example:
$arr = array( "one" => 1, "two" => 2, "three" => 3, "seventeen" => 17 ); foreach ($arr as $key => $value) { echo "Key: $key | Value: $value \n"; }
Output:
Key: one | Value: 1 Key: two | Value: 2 Key: three | Value: 3 Key: seventeen | Value: 17
Note: foreach works only on arrays and objects, and will issue an error when you try to use it on a variable with a different data type or an uninitialized variable.
WHILE
Reference Material: http://php.net/manual/en/control-structures.while.php
It is best used for array based loops that are from a database. For example:
$conn = mysql_connect("localhost", "mysql_user", "mysql_password"); mysql_select_db("mydbname"); $sql = "SELECT userid, fullname, userstatus FROM sometable WHERE userstatus = 1"; $result = mysql_query($sql); while ($row = mysql_fetch_assoc($result)) { echo $row["userid"]; echo $row["fullname"]; echo $row["userstatus"]; }
Output would be dependent on what is in “sometable”. But you get the point.
While you could use each loop a different way, using these ways should use less resources when dealing with a large amount of data. It is also good code to use the control structures the way they were intended to be used.
Please note that you will want to not write code exactly as above due to not showing error handling in these examples, especially the WHILE example.
More reference material to look at:
All Control Structures: http://php.net/manual/en/language.control-structures.php
do-while (same a while except will execute first before evaluating): http://php.net/manual/en/control-structures.do.while.php
I hope to bring out more examples soon with coding in PHP, and maybe even have a whole section of tutorials. Until then, php.net is the best resource on learning everything about php.
~Joshua